Just updated my virtual computer running Win10. Yes, I am a chicken and have not upgraded my computer from 8.1 yet. Some time in the future I will find time to upgrade, however in the mean time I am quite happy with just running it as a VM.
My current build of powershell is 10041 (yes, I know I am falling behind). I was tweeting with Jan Egil (@JanEgilRing). He mentioned a new cmdlet and a couple of new common parameters that has become available in this build.
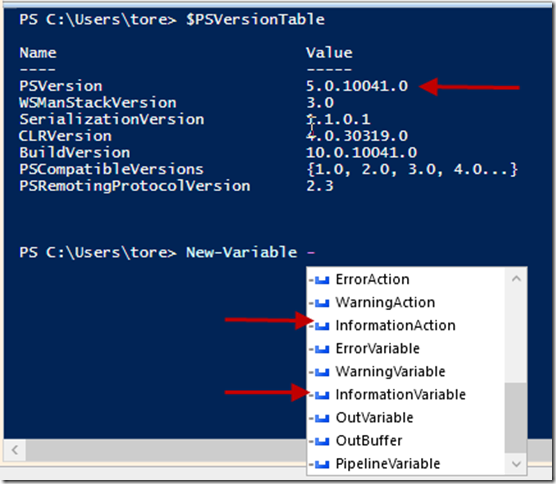
We have two new common parameters:
Using my previous get all common parameters, we can indeed confirm that these are new common parameters:
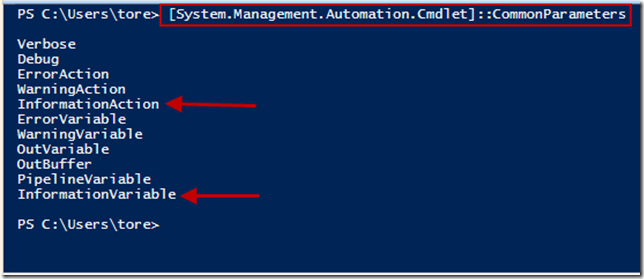
There is also a new cmdlet called Write-Information which has 2 parameters:
There is a new $InformationPreference global variable set to “Continue” by default.
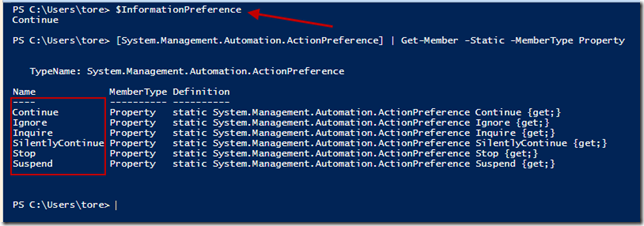
So here is the first attempt to write something in the console:
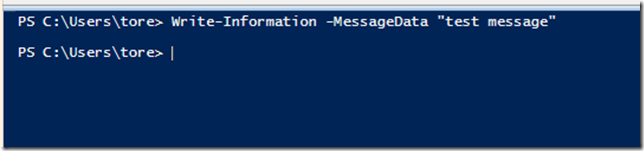
Write-Information produced no information in the console. Turns out it is not a bug, however a feature. The output/stream from the cmdlet is filtered out by the console and not shown by default. Before we get into a little bit more advanced stuff, remember that InformationVariable is a new common parameter for all cmdlets and advanced functions.
Glad you asked. Turns out there is a couple of nice use cases for this. First we need to get out hands on that information stream, it has to be there somewhere:
(Get-PowershellProcess)
What happens if we run Get-PowershellProcess function and assign the InformationVariable to a variable:
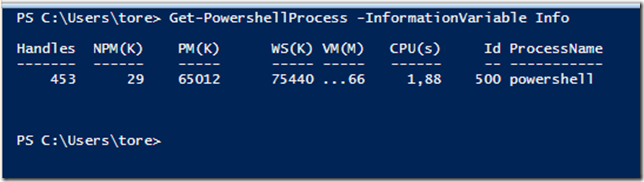
Nothing gets written to the console, however what is the content of our $Info variable?
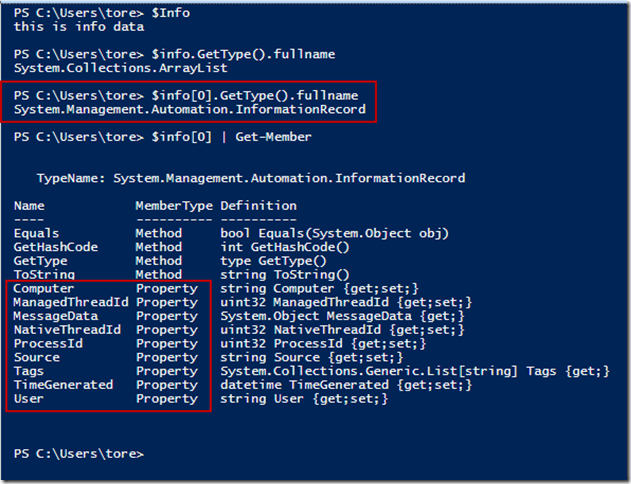
A couple of things to note here. First off the $Info variable is an ArrayList which makes sense. Secondly each item is of type InformationRecord. It is not an new type, it has been around since powershell 2.0, however it has changed a bit. Currently it supports these properties:
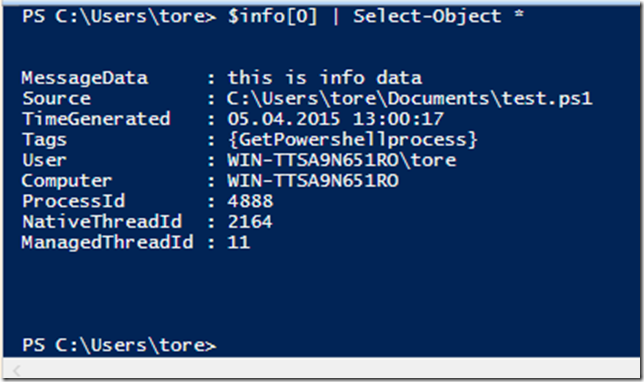
This is the InformationRecord produced by our single write-information line. We get the time it was generated, the source (script) where it originated from, the user, computer and thread information for free. In addition the tags will be stored in the tags property as an collection and finally the messagedata is stored in the messageData property.
Now just for the fun of it, lets change our function to include a Write-Host statement.
(Get-PowershellProcess with write-host)
If you check the info variable:
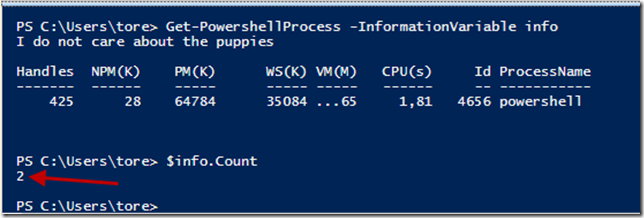
That is interesting. We now have to (2) informationRecords in our information variable.
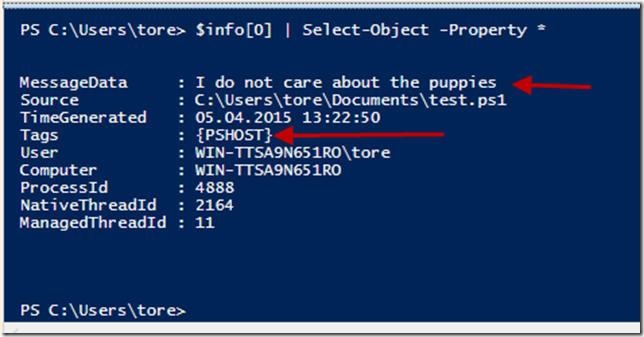
Yes, pigs are flying here. The Write-Host cmdlet is now producing output by writing to the information stream. Things to note here is that the messagedata contains the object/text you wrote using write-host. Secondly every Write-Host statement is tagged with PSHOST.
If you don’t know about streams and redirect in powershell, June Blender has written a nice post about it on the Scripting Guy’s blog, link here. With the release of WMF5 this will have to be updated since the information stream is number 6, previously we had 5:
$outputWithStreams = Get-Powershellprocess 6>&1
This will capture all output and the information stream including write-host "output".
On the top of my head this can be used in a couple of scenarios. First and foremost I will probably be using it for extra verbose(/information logging and with the tags option, I can really do filtering on the records being produced by the Write-Information cmdlet.
Secondly if you combine the output of Write-Information with the Export-CliXML cmdlet, you could build a “log-file” that contains real objects that you can import with Import-CliXML and filter the result.
Thirdly it makes no sense in not creating advanced powershell functions since this new powerful feature will be available for those. Start adding [cmdletBinding()] to your functions with an Param() block (yes it can be empty) and you are ready to go. If you are on twitter and missed the first #PSblogWeek you should look it up and see all the help the community and MVP's are producing to help you write advanced functions and help in powershell.
Fourthly I will most likely move my excess verbose logging over to Write-Information due to the increased possibility of filtering using tags and datetime.
If you have been following the debate around use/do not use Write-Host because it kills puppies, this looks like a nice save by Microsoft and the powershell team. I really like the possibility to tag the information stream and later us it for filtering. Also I appreciate the source and timegenerated properties that will help if you are chasing bugs in a module or big script.
This will also most likely replace many of the "logging-modules/functions" people have been using since it is normally more convenient to use built-in stuff than to roll your own tool.
Even if Write-Host now writes to the information stream, I think you should still avoid using it in your scripts and relay on all the other streams to produce logging, verbose, warning and error records. My worry is that this most likely will not convince people to change the default behavior of using Write-Host for feedback to the console user. Time will show, however we still need to educate current and new users of the powershell kingdom to embrace the possibilities lying before their feet.
Cheers
Tore
My current build of powershell is 10041 (yes, I know I am falling behind). I was tweeting with Jan Egil (@JanEgilRing). He mentioned a new cmdlet and a couple of new common parameters that has become available in this build.
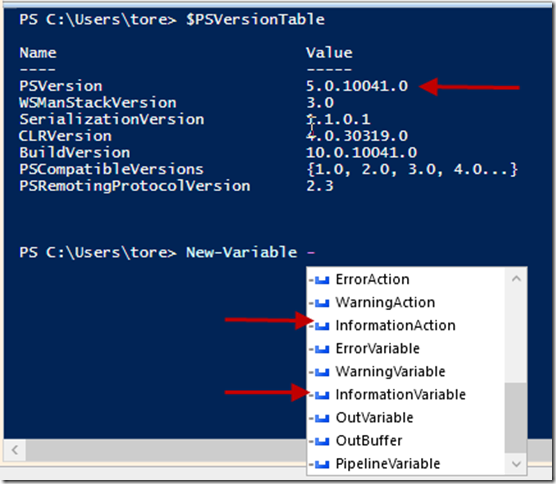
We have two new common parameters:
- InformationAction
- InformationVariable
Using my previous get all common parameters, we can indeed confirm that these are new common parameters:
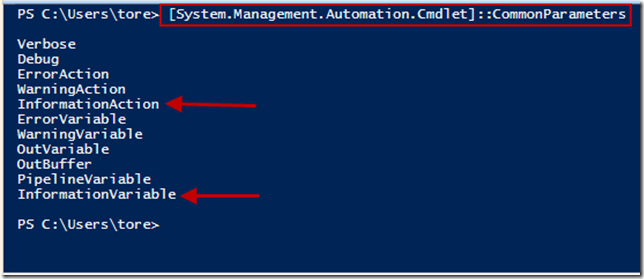
There is also a new cmdlet called Write-Information which has 2 parameters:
- [object]MessageData
- [string[]]Tags
There is a new $InformationPreference global variable set to “Continue” by default.
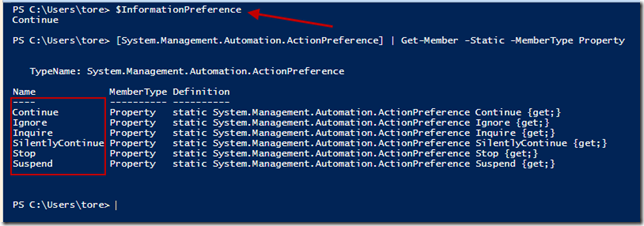
In action
So here is the first attempt to write something in the console:
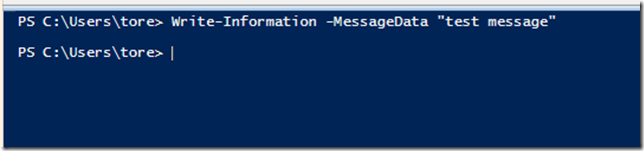
Write-Information produced no information in the console. Turns out it is not a bug, however a feature. The output/stream from the cmdlet is filtered out by the console and not shown by default. Before we get into a little bit more advanced stuff, remember that InformationVariable is a new common parameter for all cmdlets and advanced functions.
How can we use InformationVariable and Write-Information?
Glad you asked. Turns out there is a couple of nice use cases for this. First we need to get out hands on that information stream, it has to be there somewhere:
(Get-PowershellProcess)
What happens if we run Get-PowershellProcess function and assign the InformationVariable to a variable:
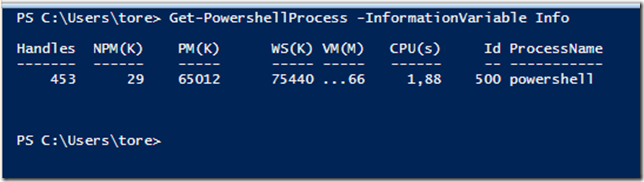
Nothing gets written to the console, however what is the content of our $Info variable?
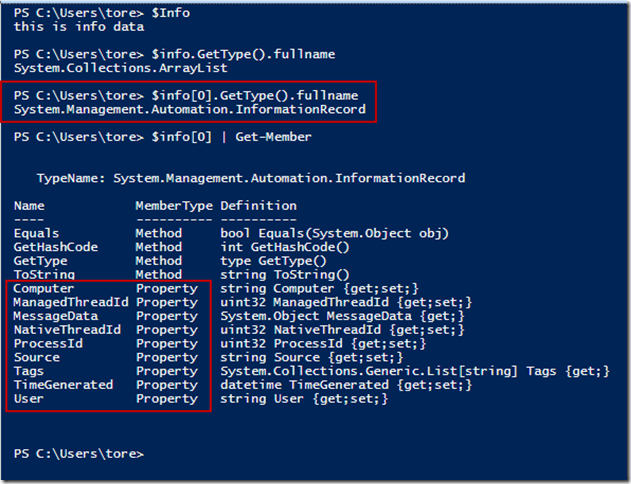
A couple of things to note here. First off the $Info variable is an ArrayList which makes sense. Secondly each item is of type InformationRecord. It is not an new type, it has been around since powershell 2.0, however it has changed a bit. Currently it supports these properties:
- Computer
- ManagedThreadId
- MessageData
- NativeThreadId
- ProcessId
- Source
- Tags
- TimeGenerated
- User
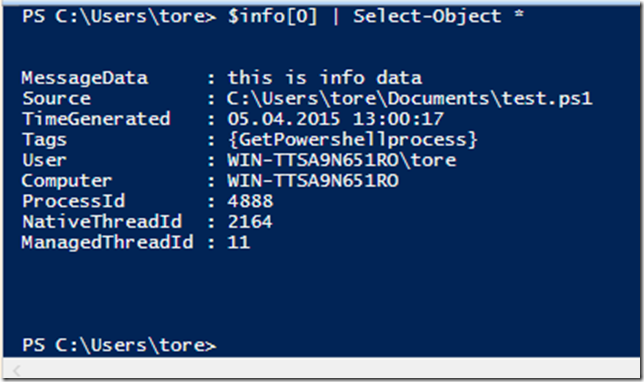
This is the InformationRecord produced by our single write-information line. We get the time it was generated, the source (script) where it originated from, the user, computer and thread information for free. In addition the tags will be stored in the tags property as an collection and finally the messagedata is stored in the messageData property.
Now just for the fun of it, lets change our function to include a Write-Host statement.
(Get-PowershellProcess with write-host)
If you check the info variable:
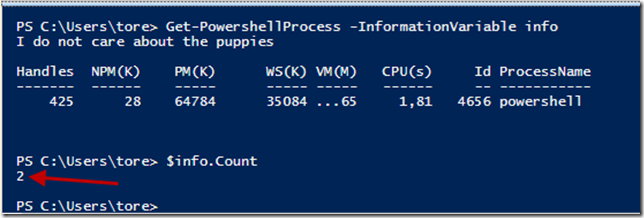
That is interesting. We now have to (2) informationRecords in our information variable.
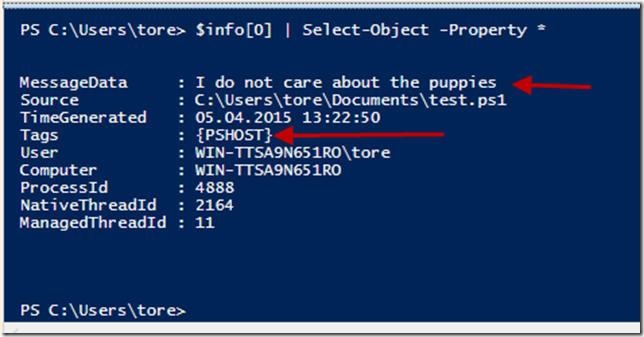
Yes, pigs are flying here. The Write-Host cmdlet is now producing output by writing to the information stream. Things to note here is that the messagedata contains the object/text you wrote using write-host. Secondly every Write-Host statement is tagged with PSHOST.
If you don’t know about streams and redirect in powershell, June Blender has written a nice post about it on the Scripting Guy’s blog, link here. With the release of WMF5 this will have to be updated since the information stream is number 6, previously we had 5:
- Output/Success
- Error
- Warning
- Verbose
- Debug
- Information
$outputWithStreams = Get-Powershellprocess 6>&1
This will capture all output and the information stream including write-host "output".
How could you use this new cmdlet
On the top of my head this can be used in a couple of scenarios. First and foremost I will probably be using it for extra verbose(/information logging and with the tags option, I can really do filtering on the records being produced by the Write-Information cmdlet.
Secondly if you combine the output of Write-Information with the Export-CliXML cmdlet, you could build a “log-file” that contains real objects that you can import with Import-CliXML and filter the result.
Thirdly it makes no sense in not creating advanced powershell functions since this new powerful feature will be available for those. Start adding [cmdletBinding()] to your functions with an Param() block (yes it can be empty) and you are ready to go. If you are on twitter and missed the first #PSblogWeek you should look it up and see all the help the community and MVP's are producing to help you write advanced functions and help in powershell.
Fourthly I will most likely move my excess verbose logging over to Write-Information due to the increased possibility of filtering using tags and datetime.
My thoughts
If you have been following the debate around use/do not use Write-Host because it kills puppies, this looks like a nice save by Microsoft and the powershell team. I really like the possibility to tag the information stream and later us it for filtering. Also I appreciate the source and timegenerated properties that will help if you are chasing bugs in a module or big script.
This will also most likely replace many of the "logging-modules/functions" people have been using since it is normally more convenient to use built-in stuff than to roll your own tool.
Even if Write-Host now writes to the information stream, I think you should still avoid using it in your scripts and relay on all the other streams to produce logging, verbose, warning and error records. My worry is that this most likely will not convince people to change the default behavior of using Write-Host for feedback to the console user. Time will show, however we still need to educate current and new users of the powershell kingdom to embrace the possibilities lying before their feet.
Cheers
Tore
Comments
Post a Comment